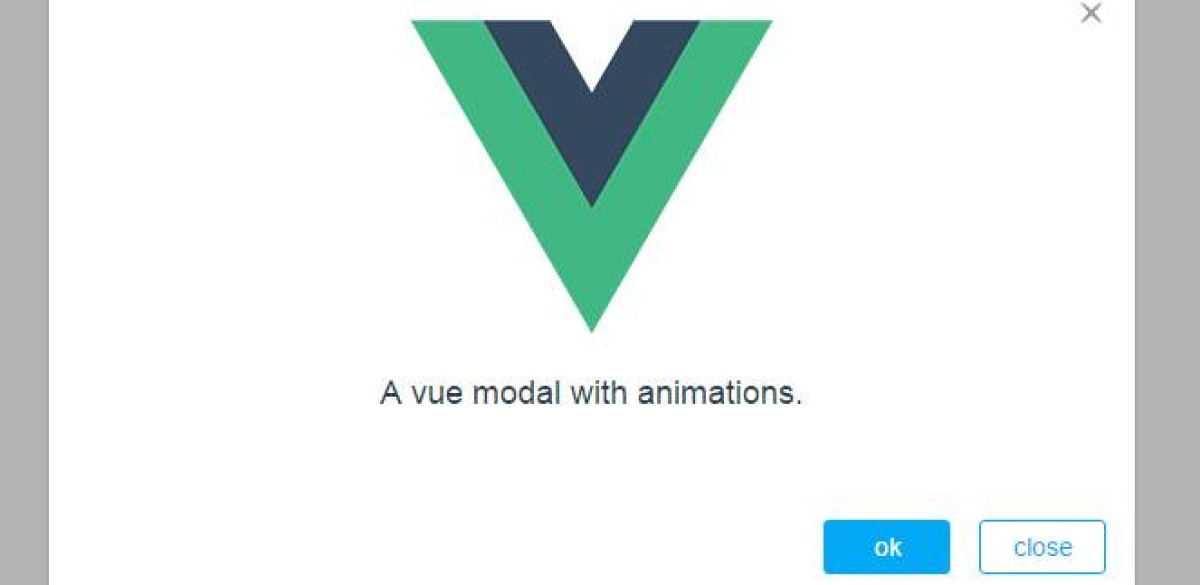
Making Self-Close Modal/Dialog in Vuejs
Importing and adapting modals and similar components to each page can be a very tedious task, especially if used frequently.
The best solution would be to access this "modal" from child "components" using a central modal (top parent). You can do this by adapting the code I shared below.
<!-- Parent Component -->
<template>
<div ref="myRef"></div>
</template>
<script>
export default {
methods:{
getMyRef(){
return this.$refs.myRef
}
},
provide() {
return{
parentRef: this.getMyRef
}
}
}
</script>
<!-- Child Component -->
<template>
<div></div>
</template>
<script>
export default {
methods:{
useRef(){
this.parentRef().data // Can access data properties
this.parentRef().method() // can access methods
}
}
inject: ['parentRef']
}
</script>
To summarize simply, the function of the code is as follows: I export (or present) the reference (myRef) that I have defined with provide (parentRef). Then I use it by importing this reference (parentRef) with inject; For example "this.parentRef().method()".
When these codes are adapted to the modal, the following result emerges. You can either close it by accessing the relevant method of the "modal" (for example, close) via reference, or you can use the related method via a button from within the component.
Other Articles
- creating-separate-env-file-for-laravel-in-localhost
- how-to-make-game-graphics-with-ai
- laravel-api-csrfxsrf-token-not-working-between-subdomain-and-domain
- react-animated-slider-react-18-nextjs-compatible
- run-nextjs-app-on-plesk
- solution-to-unity-ads-401-your-app-includes-non-compliant-sdk-version-unity-ads-on-google-play-console-issue